Hi In this post I will add example code for how to add actions in your local notification and than add their click listener.
e.g in image below where notification has two options confirm or cancel and you want to check if user has canceled or confirmed without launching application. Than follow this tutorial.
I will add separate listener for Notification click and separate for notification action button click.Step 1: Add method for creating push notification.private void sendNotification(String messageBody) { Intent intent = new Intent(this, SplashActivity.class); intent.putExtra("fromNotification", "book_ride"); intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP); Intent intentConfirm = new Intent(this, NotificationActionReceiver.class); intentConfirm.setAction("CONFIRM"); intentConfirm.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP); Intent intentCancel = new Intent(this, NotificationActionReceiver.class); intentCancel.setAction("CANCEL"); intentCancel.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TOP); //This Intent will be called when Notification will be clicked by user. PendingIntent pendingIntent = PendingIntent.getActivity(this, 0 /* Request code */, intent, PendingIntent.FLAG_ONE_SHOT); //This Intent will be called when Confirm button from notification will be //clicked by user. PendingIntent pendingIntentConfirm = PendingIntent.getBroadcast(this, 0, intentConfirm, PendingIntent.FLAG_CANCEL_CURRENT); //This Intent will be called when Cancel button from notification will be //clicked by user. PendingIntent pendingIntentCancel = PendingIntent.getBroadcast(this, 1, intentCancel, PendingIntent.FLAG_CANCEL_CURRENT); Uri defaultSoundUri = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION); NotificationCompat.Builder notificationBuilder = new NotificationCompat.Builder(this) .setSmallIcon(R.drawable.logo_steer) .setContentTitle("Madaar Ride") .setContentText("" + messageBody) .setAutoCancel(true) .setSound(defaultSoundUri) .setContentIntent(pendingIntent); notificationBuilder.addAction(R.drawable.ic_check_black, "Confirm", pendingIntentConfirm); notificationBuilder.addAction(R.drawable.ic_clear_black, "Cancel", pendingIntentCancel); NotificationManager notificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE); notificationManager.notify(11111 /* ID of notification */, notificationBuilder.build()); }
Step 2: Now Declare BroadcastReceiver for handling action events in manifest file.<receiver android:name="NotificationActionReceiver"> <intent-filter> <action android:name="CONFIRM" /> <action android:name="CANCEL" /> </intent-filter> </receiver>
Step 3: Add code for BroadcastReceiver ClassAuthor:/** * Created by hammadtariq on 8/9/2016. */ public class NotificationActionReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { if (intent.getAction().equalsIgnoreCase("CONFIRM")) { Toast.makeText(context, "Booking your ride", Toast.LENGTH_SHORT).show(); } else if (intent.getAction().equalsIgnoreCase("CANCEL")) { NotificationManager notificationManager = (NotificationManager) context.getSystemService(Context.NOTIFICATION_SERVICE); notificationManager.cancel(11111); } } }
Hammad Tariq
Android Application Developer
Thursday, October 20, 2016
android notification action button listener
Subscribe to:
Post Comments (Atom)
Kotlin Android MVP Dagger 2 Retrofit Tutorial
http://developine.com/building-android-mvp-app-in-kotlin-using-dagger-retrofit/
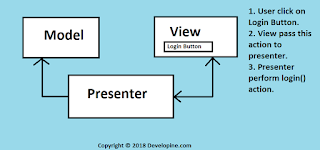
-
Hi In this post I will add example code for how to add actions in your local notification and than add their click listener. e.g in image...
-
In this example I will show you how to get precise call state in Android programatically using java reflection API. Add this in Andro...
-
Hello Guys, Now I have my own Blog Site . please visit Link for more Android and Kotlin Tutorials. In this example I will teach yo...
when i click confirm button how to navigate to particular activity
ReplyDeleteThank you for this information, it is very informative and i want to share a game. here is the link https://apkstreet.com/roblox-mod-apk-unlimited-robux
ReplyDeleteReally amazing information. I also want to share a game with you. here is the link https://apkedge.net/zombie-catchers-mod-apk/
ReplyDeleteTitanium Art® | Ti-TiTi Alloy Art - iTanium Art
ReplyDeleteTitanium Art®. TITanium damascus titanium Art®. TITOME titanium carabiners AIM MULTIPLAYER GIGATANTI (9). titanium rod in leg Color. titanium ore 7.00.
It's late finding this act. At least, it's a thing to be familiar with that there are such events exist. I agree with your Blog and I will be back to inspect it more in the future so please keep up your act. Tipnovel
ReplyDelete